Hello friends,
There comes new features and Android Nougat 7.1 is no different. One of these new features is App Shortcuts. App Shortcuts allow the user to access primary actions in your app straight from the launcher, taking the user deep into your application, by long pressing on your app icon.
Main point of shortcut use..
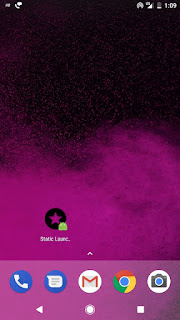
There comes new features and Android Nougat 7.1 is no different. One of these new features is App Shortcuts. App Shortcuts allow the user to access primary actions in your app straight from the launcher, taking the user deep into your application, by long pressing on your app icon.
Main point of shortcut use..
- App Shortcuts are great for exposing actions of your app and bring back users into your flow
- They can be static or dynamic
- Static are set in stone once you define them (you can only update them with an app redeploy)
- Dynamic can be changed on the fly
- You can create a back stack of activities once you open one through a shortcut
- Shortcuts can be reordered, but only in their respective type and static shortcuts will come always at the bottom as they're added first (there's no rank property to be defined on them)
- The labels of the shortcuts are CharSequence so you can manipulate them through spans.
Android app shortcut basically two type-
1. Static shortcut
2. Dynamic shortcut
How to add static shortcut:
First you create a new project or existing project go to android manifest.xml and add below line
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="samset.appshortcut">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
<meta-data
android:name="android.app.shortcuts"
android:resource="@xml/shortcut" />
</activity>
</application>
</manifest>
Now you create in a xml folder in res folder and create file shortcut.
shortcut.xml
<?xml version="1.0" encoding="utf-8"?>
<shortcuts xmlns:android="http://schemas.android.com/apk/res/android">
<shortcut
android:enabled="true"
android:icon="@drawable/ic_stars"
android:shortcutDisabledMessage="@string/disabled_message"
android:shortcutId="id_static"
android:shortcutLongLabel="@string/static_label"
android:shortcutShortLabel="@string/launcher_label">
<!-- You see here i define two intent i think you confuse why i use two intent so i confirm first intent use when your app launch and you press back then you come on MainActivity-->
<intent
android:action="android.intent.action.Main"
android:targetClass="samset.appshortcut.MainActivity"
android:targetPackage="samset.appshortcut" />
<!-- Second intent use when you long press and open shortcut then you redirect your target actvity(StaticActivity)-->
<intent
android:action="android.intent.action.VIEW"
android:targetClass="samset.appshortcut.StaticShortcutActivity"
android:targetPackage="samset.appshortcut" />
</shortcut>
<shortcut
android:enabled="true"
android:icon="@drawable/ic_settings"
android:shortcutDisabledMessage="@string/disabled_message"
android:shortcutId="id_setting"
android:shortcutLongLabel="@string/setting_label"
android:shortcutShortLabel="@string/launcher_label_settings">
<intent
android:action="android.intent.action.Main"
android:targetClass="samset.appshortcut.MainActivity"
android:targetPackage="samset.appshortcut" />
<intent
android:action="android.intent.action.VIEW"
android:targetClass="samset.appshortcut.StaticShortcutActivity"
android:targetPackage="samset.appshortcut" />
</shortcut>
</shortcuts>
- enabled: as the name states, whether the shortcut is enabled or not. If you decide to disable your static shortcut you could either set this to false, or simply remove it from the <shortcuts> set
- icon: the icon shown on the left hand side of the shortcut. In my case, I created a simple Vector Drawable from within Android Studio and assigned it as an icon
- shortcutDisabledMessage: when you disable your shortcut, it will disappear from the ones that the user can reveal by long pressing your application icon, but one can pin a shortcut to the launcher (by long pressing and dragging it on the desired launcher page), so when disabled the pinned shortcut will appear greyed out and upon tapping it a Toast with this message will appear
- shortcutLongLabel: longer text of the shortcut shown when the launcher has enough space
- shortcutShortLabel: a concise description of the shortcut. The field is mandatory. Most probably this will be the one which will appear on your launcher
- intent: here you define your intent (or more intents) that your shortcut will open upon being tapped
How to add dynamic shortcut:
MainActivity.java
package samset.appshortcut;
import android.annotation.SuppressLint;
import android.annotation.TargetApi;
import android.content.Intent;
import android.content.pm.ShortcutInfo;
import android.content.pm.ShortcutManager;
import android.graphics.drawable.Icon;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import java.util.Arrays;
public class MainActivity extends AppCompatActivity {
private ShortcutManager shortcutManager;
@SuppressLint("NewApi")
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
shortcutManager = getSystemService(ShortcutManager.class);
if (Build.VERSION.SDK_INT >= 25) {
createShorcut();
}
}
@TargetApi(25)
private void createShorcut() {
ShortcutInfo webshart = new ShortcutInfo.Builder(this, "shortcutweb")
.setShortLabel("web")
.setLongLabel("Website")
.setIcon(Icon.createWithResource(this, R.drawable.ic_touch_app))
.setIntent(new Intent(Intent.ACTION_VIEW, Uri.parse("http://samsetdev.blogspot.in/")))
.setRank(0)
.build();
ShortcutInfo dynamicShortcut = new ShortcutInfo.Builder(this, "dynamicshortcut")
.setShortLabel("Dynamic")
.setLongLabel("Dynamic Activity")
.setIcon(Icon.createWithResource(this, R.drawable.ic_touch_app))
.setIntents(new Intent[]{new Intent(Intent.ACTION_MAIN, Uri.EMPTY, this, MainActivity.class).setFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK),
new Intent(DynamicActivity.ACTION)
})
.setRank(1)
.build();
shortcutManager.setDynamicShortcuts(Arrays.asList(webshart, dynamicShortcut));
}
@TargetApi(25)
private void removeShorcuts() {
ShortcutManager shortcutManager = getSystemService(ShortcutManager.class);
shortcutManager.disableShortcuts(Arrays.asList("shortcut1"));
shortcutManager.removeAllDynamicShortcuts();
}
}
add some line of code in manifest file:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="samset.appshortcut">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
<meta-data
android:name="android.app.shortcuts"
android:resource="@xml/shortcut" />
</activity>
<activity
android:name=".StaticShortcutActivity"
android:label="@string/static_label" />
<activity
android:name=".DynamicActivity"
android:label="@string/dynamic_label">
<intent-filter>
<action android:name="samset.appshortcut.DYNAMIC_SHORTCUT" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
</activity>
</application>
</manifest>
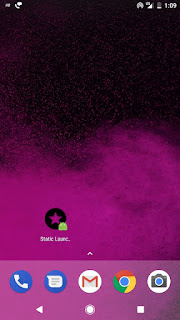
Thank you
FullSourcecode